1. Introduction
This document is intended for Techila Distributed Computing Engine End-Users who are interested in running Simulink models in parallel using TDCE. If you are unfamiliar with the terminology or the operating principles of the Techila Distributed Computing Engine technology, information on these can be found in Introduction to Techila Distributed Computing Engine.
1.1. Model Calibration
Model calibration can be a computationally intensive process that requires evaluating the model performance with several combinations of input arguments and comparing the model’s performance against real-world measurement data. In situations where there a several parameters and hundreds of different potential combinations, performing an exhaustive search over the entire parameter range can be computationally intensive and sometimes lead to making compromises on the number of parameter combinations used the calibration.
Techila Distributed Computing Engine enables running Simulink models as standalone executables on hundreds of computational nodes simultaneously. Each model can be run by using a different input parameter combination, which greatly reduces the required wall clock time when analyzing wide ranges of parameter combinations.
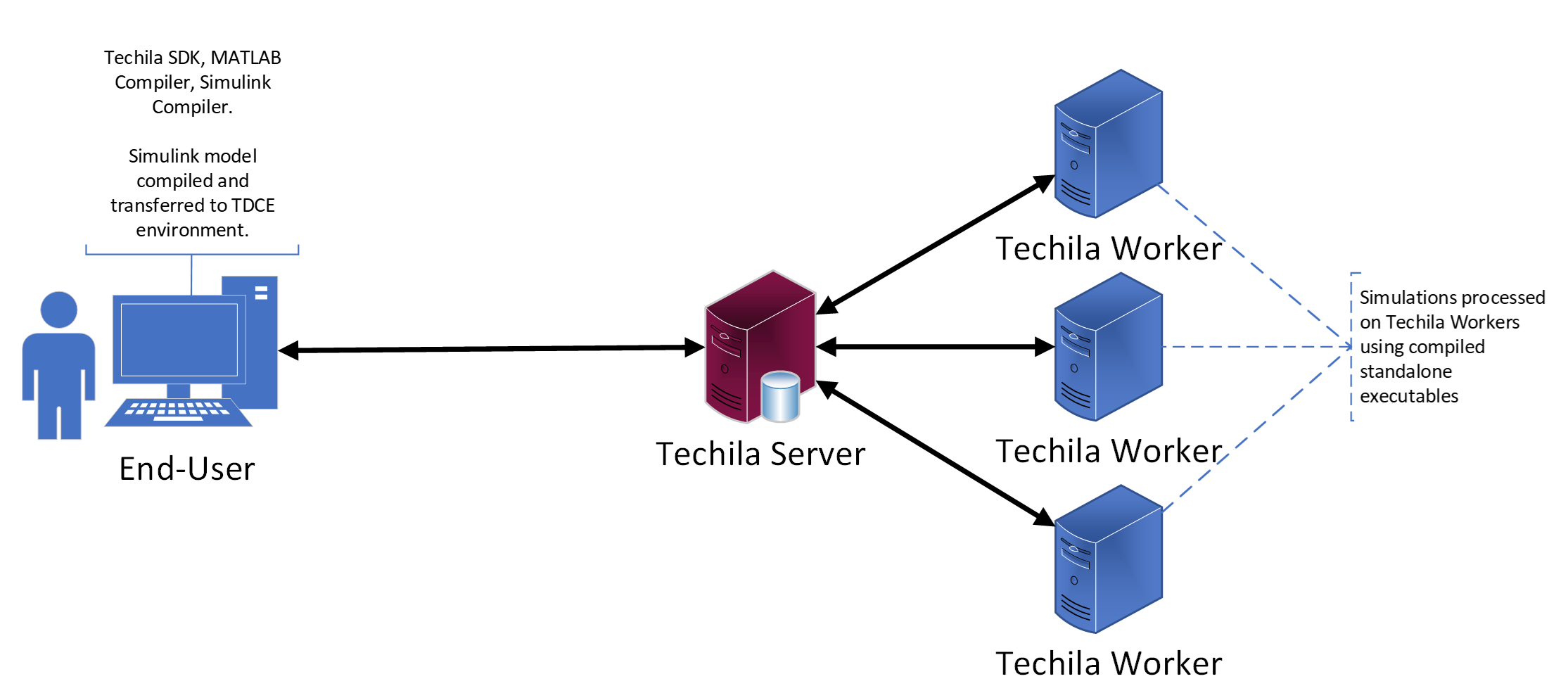
When using TDCE, parameter sweeps can be done by using the standard cloudfor
helper function that is included in the Techila SDK.
2. Configuration
2.1. MATLAB Configuration
This Chapter contains information of the preparation steps required before you can access the Techila Distributed Computing Engine environment from your MATLAB. These steps include:
2.1.1. Modifying the MATLAB Search Path
Before proceeding, please add the folder containing the Techila Distributed Computing Engine MATLAB functions to the MATLAB search path. This can be done by following the steps listed below.
-
Change your working directory in MATLAB to the following directory
<full path>\techila\lib\Matlab
-
Execute the following command:
installsdk
Additional information about modifying the MATLAB search path can be accessed with the following MATLAB commands:
doc userpath
doc startup
doc addpath
2.1.2. Testing Network Connectivity
In order to connect to your Techila Distributed Computing Engine environment, your computer must be able to establish a network connection to the Techila Server. To test that a network connection can be established, execute the following command in your MATLAB:
techilainit
If the network connection works, the function should return value 0
. Any other return value is an indication of a problem. The screenshot below shows what the output looks like when the network connection works ok.
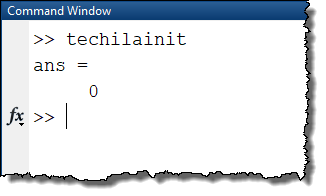
2.1.3. Configuring the Compiler
In order to process Simulink models in TDCE, the models will need to be compiled in to standalone executables, which requires the following MATLAB components:
-
MATLAB Compiler (https://www.mathworks.com/products/compiler.html).
-
Simulink Compiler (https://www.mathworks.com/products/simulink-compiler.html)
When the above components are available, you can setup your MATLAB compiler by executing the following command in MATLAB:
mbuild -setup
The screen capture below illustrates what the output of command looks like when a compiler was successfully configured:
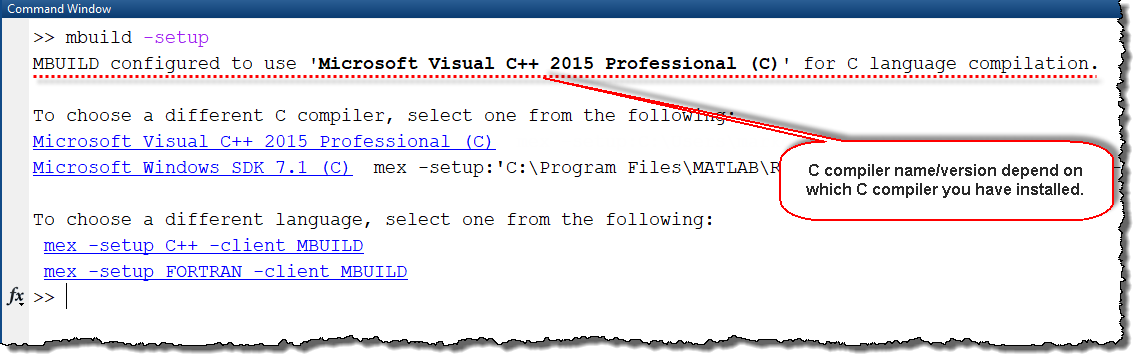
Please note that compiled binaries are platform specific and can only be executed on Techila Workers with a compatible platform according to the table below.
Compilation Platform and MATLAB Version | Compatible Techila Worker Platforms |
---|---|
Windows, 64-bit MATLAB |
Windows 64-bit |
Linux, 64-bit MATLAB |
Linux 64-bit |
2.2. Simulink Configuration
To perform parameter sweeps using Simscape models, Simulink and Simscape settings will need to configured to support running as standalone executables used for parameter sweeping. The steps below describe the required modifications.
-
If using Simscape, enable Simscape runtime parameters. This is a one-time configuration change that will allow parameters to be changed during runtime, which is required in order to perform parameter sweep type computations. More information can be found on the MathWorks documentation
-
Enable runtime parameters in the blocks containing parameters you want to sweep. This will allow you to change the model parameters when the code is executed. More information can be found in the MathWorks documentation
-
In Simulink, under Simulation settings, set the mode to
Rapid Accelerator
. This mode is required in order to run the model as a standalone executable. More information about Simulink compiler can be found in the MathWorks documentation. Additional information about how the `Rapid Accelerator works can be found in the MathWorks documentation. -
As computations will be run using
Rapid Accelerator
mode, Simscape logging will need to be disabled for the model. More information about logging settings can be found in MathWorks documentation. -
Add
sink
blocks to save signals to the workspace as workspace variables. These variables will be returned from each computational Job and will be available in your local MATLAB session after the computations are completed.
3. Examples
This Chapter contains walkthroughs of code samples that demonstrate how you can modify an existing Simulink model to perfrom parameter sweeping
3.1. Example - RC Circuit
This example is based on the MathWorks RC circuit example and shows the necessary modifications required in order to run Simscape models in TDCE and perform parameter sweeps.
Before running this example, please make sure that you have enabled Simscape to use run-time parameters as described in the first step in chapter Simulink Configuration.
-
To start, set your current working directory to the following folder:
techila\examples\Matlab\Simulink\1_rc_circuit
-
Run the
init_rc.m
script. This script will copy the RC Circuit Simulink example to the current working directory, under namemy_ssc_rc_circuit_sl.slx
. This script is shown below for reference.clear [sample_dir,~,~]=fileparts(mfilename('fullpath')); cd(sample_dir) orig_mdl = 'ssc_rc_circuit_sl.slx'; mod_file = 'my_ssc_rc_circuit_sl.slx'; if ~isfile(mod_file) copyfile(which(orig_mdl),mod_file) end [~,mod_mdl,~]=fileparts(mod_file); isModelOpen = bdIsLoaded(mod_mdl); open_system(mod_mdl);
-
Using Simulink, modify the model as described below:
-
Under Simulation settings, set the mode to
Rapid Accelerator
. -
Open the
R1
block and set the parameter toRun-time
. -
Open the
Model Settings
, selectSimscape
and set the logging toNone
. -
Add a
Sink
block and connect it the to the scope measuring the output.
-
-
Save the model.
-
Run the
run_rc_demo.m
script. This will create the computational project and return results toout
variable. After the results have been downloaded, the outputs will be visualised in a graph. This script is shown below for reference.R=10; % Required for compilation % Define sweep range R_var = 5:15; numSims = length(R_var); % Results will be stored in 'out' out = cell(1,numSims); % Run computations in TDCE cloudfor idx=1:length(R_var) if isdeployed in = Simulink.SimulationInput('my_ssc_rc_circuit_sl'); % Specify a different R value for each simulation. in = in.setVariable('R', R_var(idx)); in = simulink.compiler.configureForDeployment(in); out{idx} = sim(in); end cloudend % Visualize results in a graph. legend_labels = cell(1,numSims); for i = 1:numSims simOut = out{i}; plot(simOut.logsout_ssc_rc_circuit_sl{1}.Values.Time,simOut.logsout_ssc_rc_circuit_sl{1}.Values.Data) legend_labels{i} = ['Run ' num2str(i)]; hold all end title('RC Circuit Demo') xlabel('Time (s)'); ylabel('Output voltage (V)'); legend(legend_labels,'Location','NorthEastOutside');